Client and Server Validated Forms in Next 15
- Published on
- Reading time
- 17 min read
Intro
After building an app recently, I'm going to share several patterns I implemented in my next few blog posts. First, I'd like to discuss form validation that leverages Zod and React Hook Form. It was my first time trying these, and they were very straight forward. At work, I use Angular and GCP full stack, so having many of those tools in a single Next codebase feels super convenient!
PS: Since authentication is serious for security, I'll mention basic security considerations along the way. Be on the lookout for a couple of spooky warnings. 👻
Sections
- Prerequisites and Setup
- Client vs. Server Validation
- Prisma Schema and the Form's Zod Validator
- Building the Form
- Submitting the Form
- Displaying the Server Error
- Wrap Up
Prerequisites and Setup
For prerequisites, be familiar with Auth.js, Next.js (app router), Tailwind, and Shadcn/ui because I won't be explaining these or the UI much. Additionally, I'll use Prisma with Postgres, Zod, and React Hook Form and will go into more detail about how each are used, so if you aren't familiar, you should be ok! Here are several references:
Everything in this post can be simulated without setting up a database. I'll explain what to do differently without one in case you only want to mimic this sign up scenario. Alternatively, you can create a local Postgres DB in a Docker container or use something like PocketBase that runs on SQLite, though the schema for Auth.js will be different.
To set up a database in Vercel's dashboard, go to your project's storage tab and click "Create Database". Here, you can find free options to deploy a Postgres DB. I'm using Neon, a popular serverless Postgres service. Vercel handles the setup so that you don't need to visit Neon's platform, but it's cool too: Neon docs. Once created, Vercel returns the env variables you need to get everything connected - just make sure you keep these secret and don't display them on the client. Place them locally in a .env.local
file and add them in your Vercel project's settings. Please include this env file in your .gitignore
and DO NOT commit these or push them to GitHub. Note that Next only bundles environment variables for the client if the name is prefaced with "NEXT_PUBLIC_", so DON'T add this and everything will be square.
Sorry to be insistent about security, but you'd be surprised how many active secrets I've extracted from folk's commit history when auditing codebases for work. As an aside, you should never place keys or webhook URLs into a static codebase or commit or publish them. Even if you commit secrets and write another commit to remove them, the secrets will be exposed in the commit history. If you commit and/or publish these, rotate the exposed secrets immediately and get new ones!
You could also filter out those commits so they no longer appear in the history. The only caveat is that there are a lot of places where that history can persist, like an employee's local clone or in some random internet archive like the Wayback Machine.
Lastly, this advice applies equally to public and private repos. Doing this when the repo is private merely kicks the risk exposure out to include whether or not you remember in 3 years if that old repo you're now considering making public for your portfolio has any secrets. Spoiler alert: you won't remember, and you'll likely pwn yourself.
Client vs. Server Validation
With server and client-side code so integrated in Next, it feels too easy to validate everything in a server action (a cloud function like GCP Cloud Run or AWS Lambda). I'm not sure if anyone else feels that way, but knowing exactly when to do stuff on the client vs. the server was the main learning curve I encountered when first learning Next a few years ago. To limit server-side calls and the time users must wait for these to resolve, Zod and React Hook Form's handleSubmit ensure as many as possible of the form's fields are valid before sending a request to the action.
For the sign up scenario I'm presenting, input characteristics that can be validated client-side are name length, email shape, or matching passwords. However, a check requiring server-side validation would be to ensure an email doesn't already have an account. In this case, a server action should handle checking for uniqueness when attempting to create the user. This pattern's strength is handling each side of validation in unison via the same schema full stack (literally the same code) and providing feedback to the user without clearing the form state. In summary, it doesn't take much complexity and provides a nice, DRY developer experience!
Prisma Schema and the Form's Zod Validator
Jumping into the code, the user schema is a slightly altered version of what is presented in Auth.js's docs for Prisma's Postgres schema. My variation simply splits name into first and last name:
model User {
id String (dbgenerated("gen_random_uuid()")) .Uuid
firstName String
lastName String
email String (map: "user_email_idx")
password String
createdAt DateTime (now()) .Timestamp(6)
updatedAt DateTime
}
Using this and the other schemas from Auth.js's Prisma-Postgres docs, I performed the migration (requires a database to be set up) and generated the Prisma Client. If not using a database, then migrating isn't necessary. You will just need to mimic the server-side email error I'll mention later and not run the prisma.user.create
function.
Next, I set up the Zod schema for the signup form. This includes messages about issues I want to prevent on the client side and will be used with React Hook Form's error checker to prevent submission if anything isn't right. Ultimately the schema below is quite readable and self-explanatory, the only bit worth mentioning is how refine works. With refine, I can cross-validate interdependent fields such as ensuring the passwords match. Error messages target the field provided in the path when an error occurs. By "target" I mean that I am specifying exactly which form input's error message I want this error to be displayed against in the UI. This will make sense after templating the form.
export const signUpSchema = z
.object({
firstName: z.string().min(2, 'First name must be at least 2 characters'),
lastName: z.string().min(2, 'Last name must be at least 2 characters'),
email: z.string().email('Invalid email address'),
password: z.string().min(12, 'Password must be at least 12 characters'),
confirmPassword: z.string().min(12, 'Password must be at least 12 characters'),
})
.refine((data) => data.password === data.confirmPassword, {
message: 'Passwords must match',
path: ['confirmPassword'],
})
Building the Form
From Shadcn/ui, I use Form
, FormControl
, FormItem
, FormLabel
, FormField
, and FormMessage
along with Button
and Input
. To add these run the following:
npx shadcn@latest init # If you need to set up shadcn
npx shadcn@latest add form input button
I'll start by templating the form to get the necessary fields and controllers from the useForm
hook. I'll explain this more below, but for now, check out the structure:
// Shadcn Component imports...
import { signUpSchema } from '@/validators'
import { useForm } from 'react-hook-form'
import { zodResolver } from '@hookform/resolvers/zod'
import { z } from 'zod'
const SignUpForm = () => {
const form = useForm<z.infer<typeof signUpSchema>>({
resolver: zodResolver(signUpSchema),
defaultValues: {
firstName: '',
lastName: '',
email: '',
password: '',
confirmPassword: '',
},
})
return (
<Form>
<form method="POST" {...form}>
<FormField
control={form.control}
name="firstName"
render={({ field }) => (
<FormItem>
<FormLabel>First Name</FormLabel>
<FormControl className="!mt-0">
<Input
id="firstName"
type="text"
required
autoComplete="given-name"
placeholder="Enter your first name"
{...field}
/>
</FormControl>
<FormMessage className="!m-0 text-end" />
</FormItem>
)}
/>
// More form fields...
</form>
</Form>
)
}
useForm
creates a form object, and it's type is inferred from the schema with <z.infer<typeof signUpSchema>>
. As for what the hook takes in, the default values are self-explanatory, though the keys must match the name attribute on each FormField. Next, the resolver's primary function is to validate and error-check the form. React Hook Form integrates with Zod so that signUpSchema can be directly used for error checking with the provided zodResolver
. This runs before form submission and works to parse and format the error messages I've written into the schema.
Regarding the template, that spread over useForm's form object onto the form tag adds event handlers like onSubmit and a ref. This ref is used internally for React Hook Form's programmatic manipulation of the form like resets or submissions. Shadcn's Form, FormItem, FromControl, and FormLabel are primarily here for accessibility and styling. Functionally, FormField and FormMessage are more important to explain. FormField links the form object's fields to each Input returned in the render callback. The field variable from this is spread onto Input to add more event handlers and the name attribute that was set on FormField. Via FormField and the zodResolver, FormMessage will be used behind the scenes to display errors.
By default, errors won't appear as the user types. If the first submission is invalid, then errors are updated on every keystroke. They will disappear when fixed, but can return if you change something to be invalid. Feel free to try this out by submitting a last name that's too short, then fix it so the error message goes away, and finally change the password confirmation so that they no longer match. The error message about matching passwords should immediately display without resubmitting.
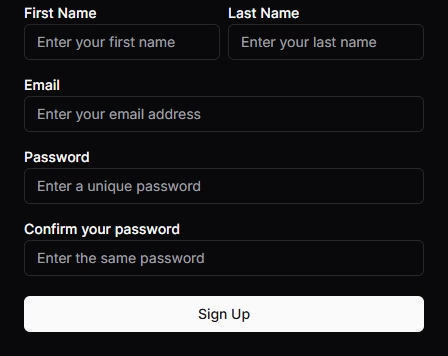
You can add additional layout styling you want like how I flexed the first and last names as show above. Otherwise, things are functionally good to go thus far! The next step is the submission handler.
Submitting the Form
For context, React Hook Form tracks validity with an isValid state, blocks submission when invalid, and aids in displaying validity errors in the correct FormMessage component. This is why it was important to set a target for confirmPassword so that the non-matching password error will display on that control's FormMessage.
Now that the validation is hooked up, the form's handleSubmit method will be used on submit to accomplish several things. handleSubmit takes in a callback function that will run if the form is valid. This callback should contain the usual async handling that an onSubmit would have like posting the data to the server, handling returned errors, creating success toasts, redirecting, revalidating, etc...
First, zodResolver runs, and the result determines if the provided callback will execute. Internally, handleSubmit uses the isValid state which is set based on what is returned from zodResolver. When invalid, in addition to preventing the callback, handleSubmit updates an internal error object used by the FormField component to display the form's errors in its corresponding FormMessage child. From the template above, this isn't very verbose, but if you check out the code for Shadcn's Form component, you can see how these interact. Here are some client error examples:
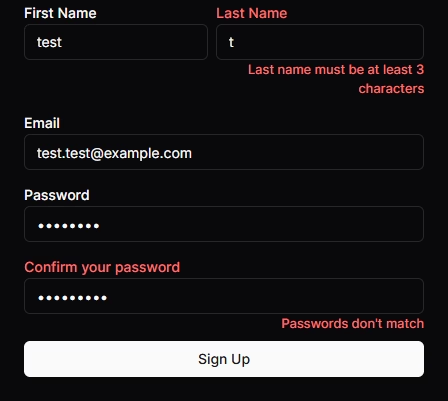
Additionally, form.formState
contains isSubmitting state that can be used to track if things are pending or not. This can be used to display loading UI so that users have clear visual feedback that something is happening while waiting for the server's response. Here are the additions to the template and the onSubmit callback:
import { SubmitHandler, useForm } from 'react-hook-form' // Added SubmitHandler
import { signUp } from '@/app/actions/user.actions.ts' // Will create this next
const SignUpForm = () => {
// Form definition remains the same...
const onSubmit: SubmitHandler<z.infer<typeof signUpFormSchema>> = async (formData) => {
try {
const res = await signUp(formData)
if (res.success) {
// Let the use know it worked! 😄
// Redirect to homepage
}
} catch (error) {
// Let the user know it failed 🥺
}
}
return (
<Form>
<form method="POST" {...form} onSubmit={form.handleSubmit(onSubmit)}>
{/* Form fields remain the same... */}
<Button
type="submit"
className="!mt-2 w-full"
variant="default"
disabled={form.formState.isSubmitting || !form.formState.isValid}
>
{form.formState.isSubmitting ? 'Creating your account...' : 'Sign Up'}
</Button>
</form>
</Form>
)
}
The formState determines if the button is disabled such as when being submitted or when awaiting for the user to correct errors. Once clicked, isSubmitting is true and the display is changed to reflect this while loading. The callback runs to post the new user's data to a server action called signUp. This action should do several things when creating the account such as salting/hashing and storing the password, but I want to focus on the validation aspect.
Primarily, user emails must be unique for every user which is something that must be checked on the server-side for security. Because our Prisma schema declares that email is @unique(...)
, the user creation can proceed as usual and the check for uniqueness will be automatically handled. However, if not unique, Prisma's error message must be parsed and returned to the client to inform the user that the account creation failed. Here is an example of how to handle this creation error and return it in the server action:
'use server'
import { signUpSchema } from '@/validators'
export async function signUp(formData: z.infer<typeof signUpFormSchema>) {
try {
// This may be redundant if you don't intend to handle errors thrown by .parse directly
const user = signUpFormSchema.parse(formData)
// Salt and hash password...
// THIS WILL THROW AN ERROR IF THE EMAIL IS NOT UNIQUE
// ---------------------------------------------------
const res = await prisma.user.create({
data: {
firstName: user.firstName,
lastName: user.lastName,
email: user.email,
password: user.password, // Salted and Hashed version
},
})
// Handle success and auto sign in after creation...
return { success: true, message: 'Sign up successful' }
} catch (error) {
let msg = (error.name === 'PrismaClientKnownRequestError' && error?.code === 'P2002')
? 'Account creation failed, please try again!'
: 'Something went wrong...'
return { success: false, message: msg }
}
}
In terms of developer experience, this code is pretty nice because it accomplishes a revalidation of every field server-side using the same zod schema used on the client. It is best practice to do this, even though the back and front end are so tightly woven together! Though not necessary, signUpSchema.parse
will throw an error that contains an array of all error messages that can be processed and returned to the user. If the form is valid from Zod's perspective, the action can call to the database via Prisma to create the user, and our Postgres schema will handle additional requirements.
For example, P2002 referenced in the catch comes from Prisma's error code documentation and means "unique constraint failed...". This is Prisma's wrapper around the Postgres unique constraint error because the database is what throws this. I know it must be related to email because the email field is the only one required to be unique. Many other errors could arise, so it would be more precise to process specific errors and show the user what you want based on whatever scenario the form is being used for. I've only differentiated these messages to show how Prisma's errors can be accessed, but both messages are intentionally vague.
This is also the spot where you would process Zod .parse errors if they show up! In this case, validation should be mostly taken care of by the front end, but in some other scenario you can process validation errors directly with:
if (error instanceof z.ZodError) {
const fieldErrorMessages = error.errors.map((err) => err.message)
}
Secure Authentication Feedback
Why didn't I directly state "Email already taken"? Not all errors should give super-specific feedback. If there are any errors involving credentials stored on the database, it's best to be vague about what went wrong. For instance, if a user submits an incorrect password to sign in, don't respond with a message that says "Incorrect password" because this implies an account with that email exists. Instead, be indirect and say something like "Incorrect credentials". This avoids addressing exactly which field was incorrect while guiding people who might've entered a typo to double-check what they've entered. It is a tricky balance between security and user experience.
In email enumeration attacks designed to gather information for phishing scams, an attacker might programmatically enter many emails, collect data about which ones exist based on feedback messages, and then use that to impersonate the owner of the website and scam that business's users. The reason this works is that scammers try and build trust through social engineering. By impersonating a business a person may expect to receive emails from, they can gain trust quickly and trick folks into forking over other personal information.
Displaying the Server Error
In the next iteration, I've added state for the error that initializes to null. This is used in the template to dynamically display an error if one is returned from the server. Immediately after the request, an error is checked which throws if res.success is false
. This starts the catch of the onSubmit where setError sets the new error message. Now error will update in the UI to show whatever message is returned from the server action. Importantly, the state of the form is not reset, so the user won't need to type in any information again.
const SignUpForm = () => {
// Form definition remains the same...
const [error, setError] = useState<string | null>(null)
const onSubmit: SubmitHandler<z.infer<typeof signUpFormSchema>> = async (formData) => {
try {
// Request remains the same...
// Should immediately follow request
if (!res.success) throw new Error(res.message)
// Handling success remains the same...
} catch (error) {
setError((error as Error).message)
}
}
return (
<Form>
<form method="POST" {...form} onSubmit={handleSubmit(onSubmit)}>
{/* Form fields remain the same... */}
{error && (
<div className="text-end text-destructive">{error}</div>
)}
{/* Submit button remains the same... */}
</form>
</Form>
)
}
Remember that the above assumes the object returned from the action always contains a success status and message text. With this in place the form with one of these errors will display like this:
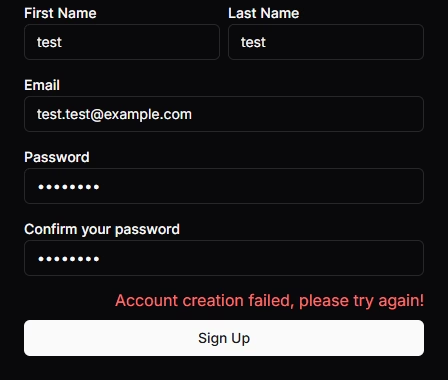
Wrap Up
That wraps up this pattern for validating forms. Remember, the focus of this post was primarily on building and validating A form, not specifically a SIGN UP form - or every facet of authenticating and creating user accounts. I didn't cover every security detail like handling passwords or securing the database, etc... For authentication, it's often better practice these days to ditch email/password altogether and use OAuth providers like Google, Microsoft, and Apple. I've added Google OAuth to this app, as made visible in the thumbnail, and it is easy to do with Auth.js providers, but that is a topic for another post.
Till the next one (which is probably going to cover database-triggered server actions), thanks for reading!